1、本例用1602液晶循环右移显示一串字符串。显示模式设置如下
1)16*2显示,5*7点阵,8位数据接口
2)开显示,有光标且光标闪烁
3)光标右移,字符不移动
2、由于一次只能向液晶写入一个字符,因此如果需要显示字符串的话,需要用到指针或者字符串数组,然后再设置一个循环,从第一个字符开始写入液晶,直到写到字符串结束标志“\0”为止。
字符显示地址的确定:由于1602液晶在第一个地址显示完毕后,能够自动指向先一个地址,因此只需要指定字符串的第一个字符显示的地址即可,
3、在keil c51中新建工程ex52,编写如下程序代码,编译并生成ex52.hex文件
//LCD1602.C
//液晶控制与显示程序
#include <reg51.h>
#include <intrins.h>
sbit RS=P2^0; //寄存器选择位,将RS位定义为P2.0引脚
sbit RW=P2^1; //读写选择位,将RW位定义为P2.1引脚
sbit E=P2^2; //使能信号位,将E位定义为P2.2引脚
//延时函数
void delayms(unsigned int ms)
{
unsigned char i;
while(ms--)
{
for(i = 0;i < 120;i++);
}
}
//忙检测函数
unsigned char busy_check(void)
{
unsigned char LCD_Status; //定义忙状态变量
RS = 0; //
RW = 1;
EN = 1;
delayms(1);
LCD_Status = P0; //读取忙状态
EN = 0;
return LCD_Status; //返回忙状态
}
//写命令
void write_LCD_Command(unsigned char cmd)
{
while((busy_check() & 0x80) == 0x80);//等待忙状态结束
RS = 0;
RW = 0;
EN = 0;
P0 = cmd;
EN = 1;
delayms(1);
EN = 0;
}
//写数据
void write_LCD_data(unsigned char dat)
{
while((busy_check() & 0x80) == 0x80);
RS = 1;
RW = 0;
EN = 0;
P0 = dat;
EN = 1;
delayms(1);
EN = 0;
}
//初始化
void init_LCD(void)
{
write_LCD_Command(0x38); //显示模式设置
delayms(1);
write_LCD_Command(0x01); //清屏
delayms(1);
write_LCD_Command(0x06); //字符进入模式:屏幕不动,字符后移
delayms(1);
write_LCD_Command(0x0c); //显示开,关光标
delayms(1);
}
//显示字符串
void ShowString(unsigned char x,unsigned char y,unsigned char *str)
{
unsigned char i = 0;
//设置起始位置
if(y == 0)
{
write_LCD_Command(0x80 | x);
}
if(y == 1)
{
write_LCD_Command(0xc0 | x);
}
//输出字符串
for(i = 0; i < 16;i++)
{
write_LCD_data(str[i]);
}
}
4、在proteus中新建仿真文件ex52.dsn,电路原理图如下所示
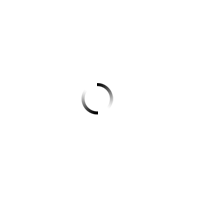
5、将ex52.hex文件载入at89c51中,启动仿真,观察运行结果。下图是程序运行结果。
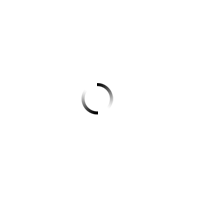